Web application development with Python Flask
Flask is one of the most popular web frameworks in Python. It is a light-weight micro-framework that makes it very easy to start writing web applications, but it is also feature rich so you can create complex applications. In this course we'll start from a basic application just to display Hello World. Then we are going to learn how to create user interaction with the back-end. We'll learn how to use both SQL and NoSQL as the back-end database of the application. By the end of the course you'll be able to create the back-end of impressive web applications.Target Audience
- Developers
- QA engineers
- DevOps engineers
Prerequisites
- At least 1 year experience programming in Python.
- Bring your own computer where you have the rights to install new software.
Objectives
- Write a web application in Python using Flask
Course Format
- 40 academic hours. (Five full days)
Language
- The course is given in Hebrew with slides and materials in English.
Syllabus
- Introduction
- Installing Flask on Linux, Windows, and Mac OSX
- Hello World with Flask
- Introduction to routes
- Accepting input from the user (GET, POST)
- Handling Query String
- Handling HTML forms
- Flexible routes to create nice URLs
- Developing Flask inside a Docker container
- Login system, sessions management, cookies
- Introduction to HTML
- Introduction to CSS
- Creating a REST API with Flask
- Returning JSON instead of HTML
- Accepting JSON as input
- Creating nice 404 page
- Returning 404 and other error codes
- Redirection
- Testing our Flask-based web application
- Using Redis as a cache
- Sending email from Flask
- Deploying a Flask based application to the cloud
- Setting up Flask with Nginx and Uwsgi
- Templates
- Introduction to the Jinja templating system of Flask
- Individual variables
- Conditionals
- Loops
- Include other templates
- Inheritance from a base template
- Relational Database (SQL)
- Quick overview
- Creating a simple schema
- Creating the database (SQLite)
- Accessing SQL database
- Inserting data into the database
- Fetching data from the database
- Updating data
- Deleting data
- NoSQL Database (MongoDB)
- Quick introduction
- Installing MongoDB
- Connecting to the database
- Inserting data into the database
- Fetching data from the database
- Updating data
- Deleting data
- Bootstrap Front-end
- Install Bootstrap
- Combining Bootstrap with our templating system
- Sample Applications
- TODO list application
- URL shortener
- An interactive game
Keywords
- python
- web
- html
- Flask
Contact
Contact: Gabor Szabo gabor@hostlocal.com
Phone: +972-54-4624648
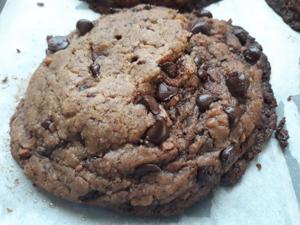